As you know, there are many programming languages that can be used in order to implement various features in a web or application, among all the languages, one of the most popular is JavaScript, many programmers use it because it can eventually be used to make the user interact with the content better which is one of the most important factors in attracting users to your websites , there are several features in this language that you can learn by mastering each of them in the best possible way, which we are going to examine variables and data types in JS.
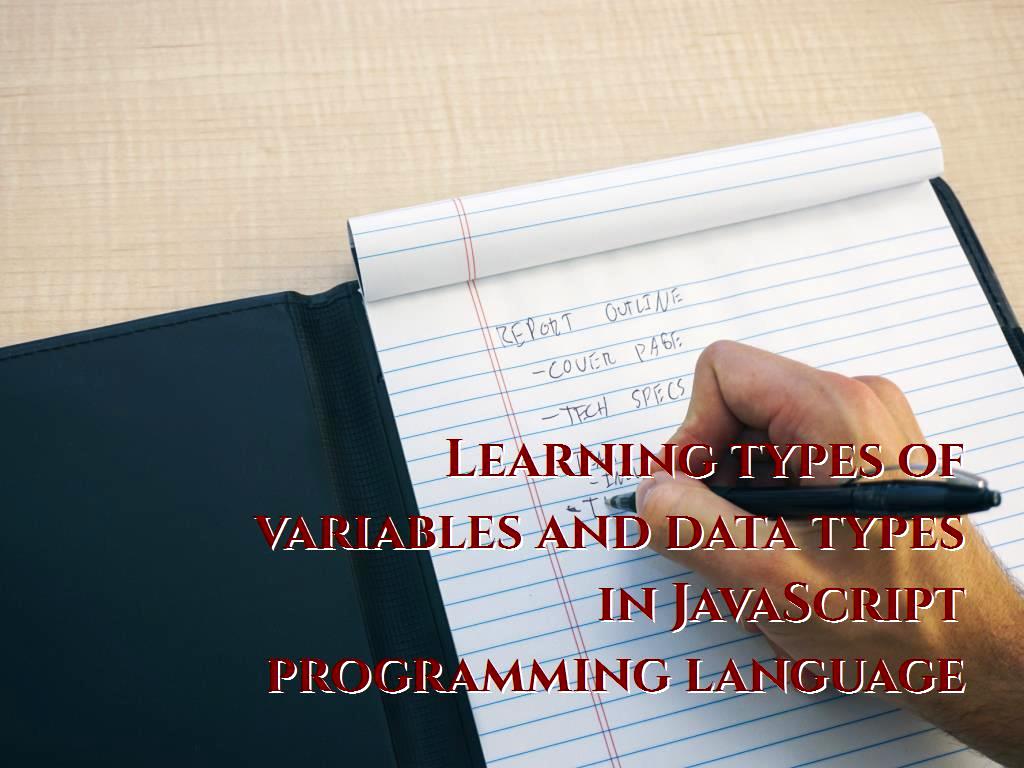
What is JavaScript?
The abbreviation for this language is JS, which allows you to use it to create dynamic content on the web, which has a very high interaction with the user, also with the help of this language, even very complex features can be easily implemented on the web, and the point that should be mentioned here is that any program written in JavaScript is known as a “script”.
JS can be run on both browsers and servers, there are JavaScript engines in various browsers, which are actually embedded by JS scripts and can be used to convert the script into a language that can be recognized by various devices.
JavaScript Variables:
In order to be able to store different amounts of data, you need to get help from it, which in order to master this, we suggest you pay attention to the following example.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p> In this example, x, y, and z are variables. </p>
<p id = "demo"> </p>
<script>
var x = 7;
var y = 8;
var z = x + y;
document.getElementById ("demo"). innerHTML =
"The value of z is:" + z;
</script>
</body>
</html>
Let and const:
The case which we have mentioned at the beginning was the only way that have been used in order to store data values until 2015, but now other ways are being used instead, including let and const.
- Const:
When you want to define a variable that cannot be changed again, you must use this.
- Let:
When the variable has a limited range, it is time to use this.
Much Like Algebra:
In this case, in order to try to store data values, you must use variables such as price1, for example.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p id = "demo"> </p>
<script>
var price1 = 4;
var price2 = 9;
var total = price1 + price2;
document.getElementById ("demo"). innerHTML =
"The total is:" + total;
</script>
</body>
</html>
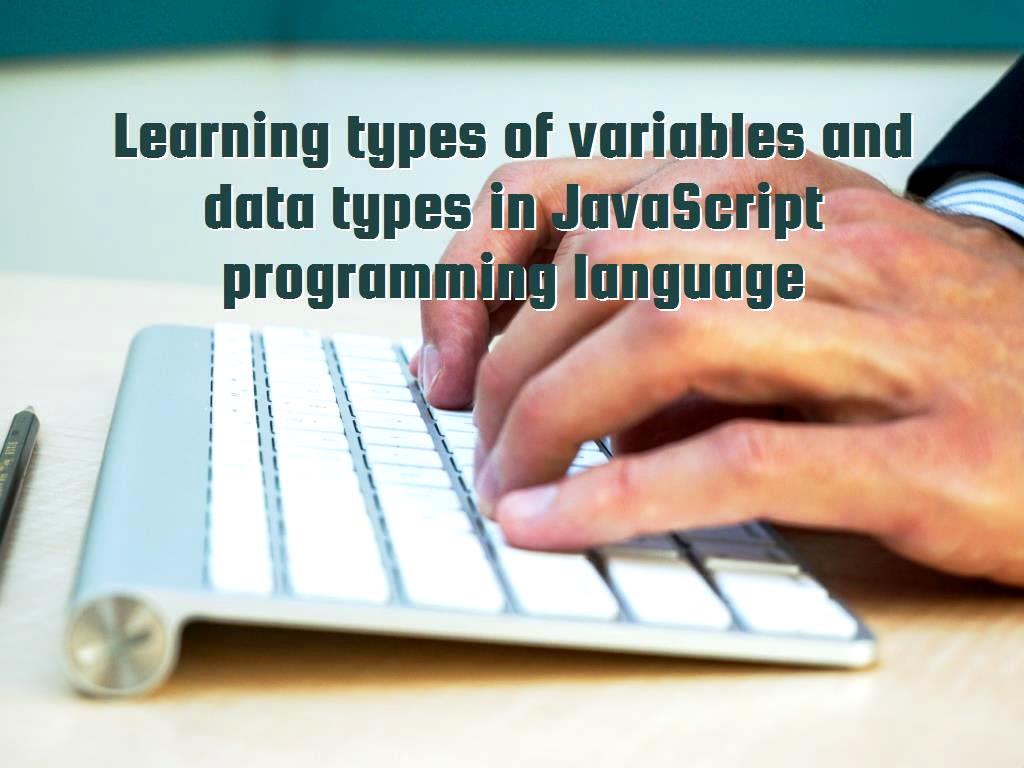
When you plan to take action in this area, you need to follow the rules, some of which we are going to explain below.
- Identifiers are very important and in fact they can be in a form of short names or descriptive names.
- While using names for identifiers, you have to pay attention to the uppercase and lowercase letters that are used in them, because even with a large and small change in one of the letters, it is considered as a different variable.
- In relation to the names which are being used, it is important that they start with a letter.
- It is possible to use Dollar Sign $ for import, now note the example below in this regard.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript $ </h2>
<p> The dollar sign is treated as a letter in JavaScript names. </p>
<p id = "demo"> </p>
<script>
var $ = 2;
var $ myMoney = 5;
document.getElementById ("demo"). innerHTML = $ + $ myMoney;
</script>
</body>
</html>
Underscore (_) may also be used to enter various data, which is mentioned in the example below.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript $ </h2>
<p> The underscore is treated as a letter in JavaScript names. </p>
<p id = "demo"> </p>
<script>
var _x = 2;
var _100 = 5;
document.getElementById ("demo"). innerHTML = _x + _100;
</script>
</body>
</html>
JavaScript Data Types:
In JavaScript , data can be in lots of different forms, they can be in letters or numbers that differ from each other, for example, when you are writing codes, quotes must be used, but you do not need to use quotes while entering numbers, if you do not pay attention to this point, the commands may not be executed correctly, for example, if you quote a number, it may behave as a text string.
It is important to note that in programming , text values are called text strings, in addition to the above, the data in JavaScript can have different types, which in this article we are going to examine the numbers and text data, which are called text strings, here note the example below.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p> Strings are written with quotes. </p>
<p> Numbers are written without quotes. </p>
<p id = "demo"> </p>
<script>
var pi = 55;
var person = "John Doe";
var answer = 'Yes I am!';
document.getElementById ("demo"). innerHTML =
pi + "<br>" + person + "<br>" + answer;
</script>
</body>
</html>
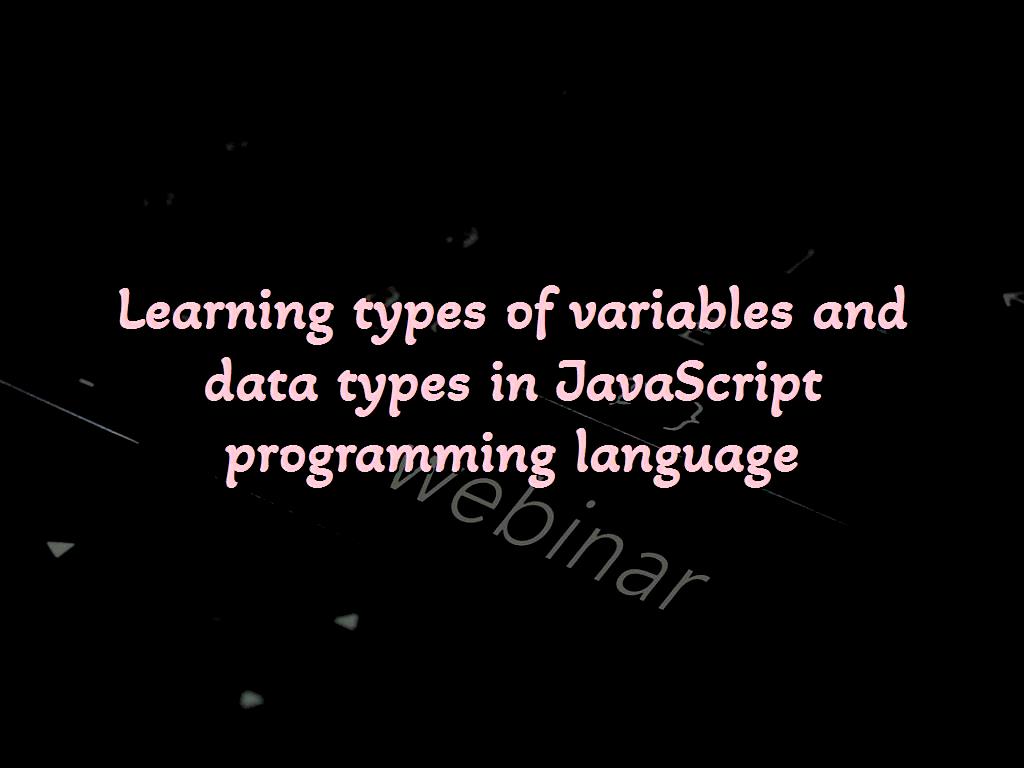
Declaring:
It is the creation of a variable in JavaScript that requires you to use the var keyword in order to declare a variable in this language, when you want to add a value to a variable, you have to do the following, in order to understand this better, pay attention to the example below.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p> Create a variable, assign a value to it, and display it: </p>
<p id = "demo"> </p>
<script>
var carName = "Volvo";
document.getElementById ("demo"). innerHTML = carName;
</script>
</body>
</html>
In addition to being able to use these features and declaring a variable, it is possible to separate the various variables you enter by using commas, now consider the following example.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p> You can declare many variables in one statement. </p>
<p id = "demo"> </p>
<script>
var person = "John Doe", carName = "Volvo", price = 200;
document.getElementById ("demo"). innerHTML = carName;
</script>
</body>
</html>
In most cases, no value is defined for the variables when they are imported, as this data may be calculated later by the users, or it may be entered at another time by the program owner, now consider the following example.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Variables </h2>
<p> A variable declared without a value will have the value undefined. </p>
<p id = "demo"> </p>
<script>
var carName;
document.getElementById ("demo"). innerHTML = carName;
</script>
</body>
</html>
In JavaScript, one variable can be used to store different types of data, here consider the following example in this regard.
<! DOCTYPE html>
<html>
<body>
<h2> JavaScript Data Types </h2>
<p> JavaScript has dynamic types. This means that the same variable can be used to hold different data types: </p>
<p id = "demo"> </p>
<script>
var x; // Now x is undefined
x = 5; // Now x is a Number
x = "John"; // Now x is a String
document.getElementById ("demo"). innerHTML = x;
</script>
</body>
</html>
It may be as follows:
- JavaScript Strings
- JavaScript Numbers
- JavaScript Booleans
- JavaScript Arrays
- JavaScript Objects
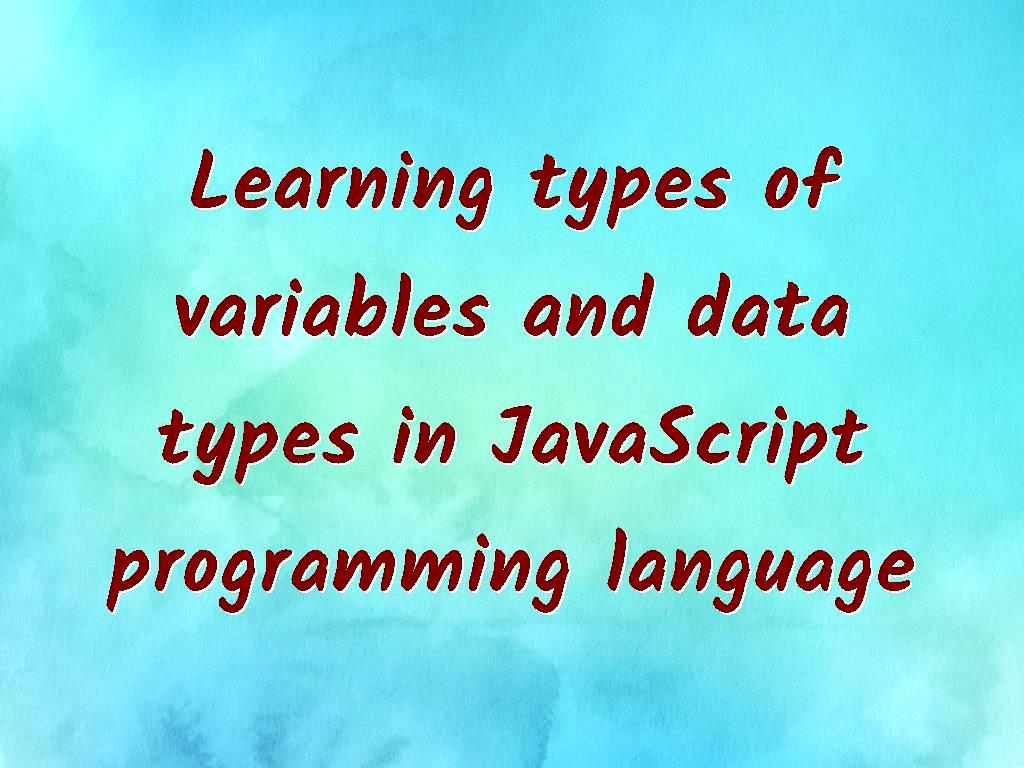
Last word:
As you can see in this article, there are different ways to store different amounts of data, and the available data may be in different forms, which we explained and gave examples in this regard, but the most important point is that you have to follow all the instructions while entering commands in order to execute properly, as a result, if you pay attention to the mentioned point as well as the examples which have been described, you can have more awareness in the field of data types and various variables as well as the way that they are being used, in this article we have explained JS programming language which is so popular among various programmers and is being used by them, we hope you can get help from this article in order to write codes in the best possible way without being wrong.