Web owners are always trying to improve the quality of content on the web and in order to achieve this goal, they take various measures and use all available facilities, to draw graphics on web pages, you can use the Canvas element, which we are going to explain to you in this regard, so that we can learn the ways to implement important things together, such as adding shadows, text, and so on.
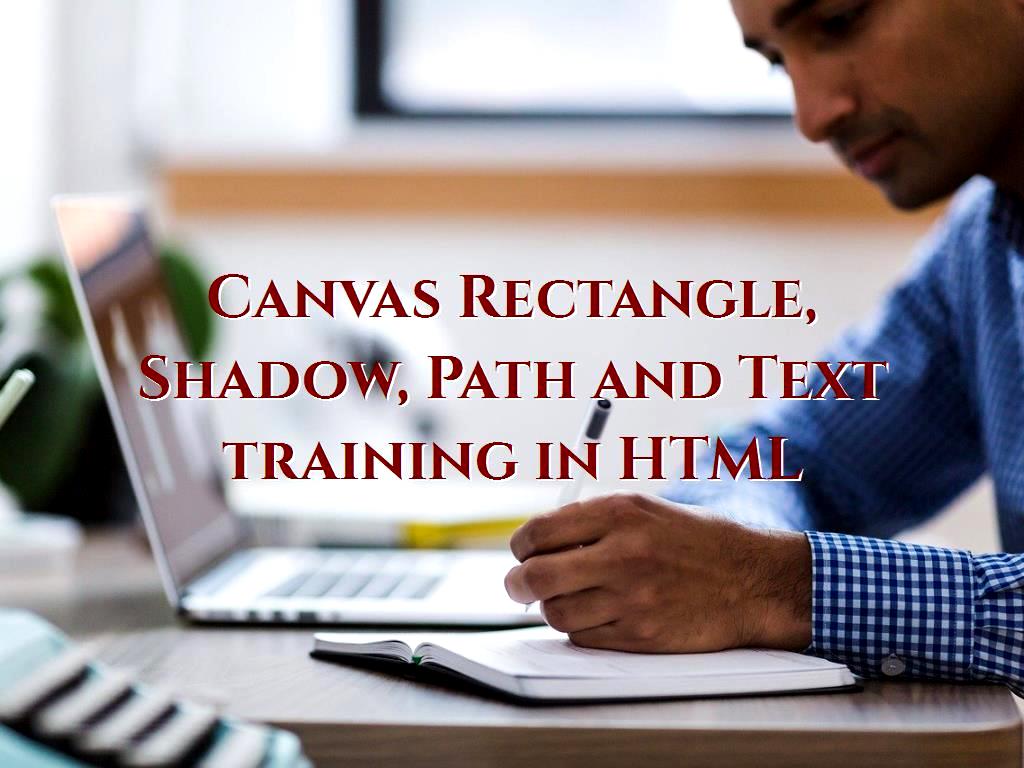
What is HTML Canvas?
This element is used to draw graphics, and the important point is that, in order to do this, scripting is required, which is usually used in JavaScript and you can apply the graphics through it, finally, with the help of this element, text, shadows, paths, etc., can be added to the images, if you want to make them more attractive to different users.
This element is one of the most attractive features in HTML5 , and it should be noted that almost all browsers today support Canvas, and through it, you can draw graphics using JavaScript while running (On The Fly).
Canvas Rectangle:
This method is used in order to draw a rectangle which has various parameters that we are going to mention in the following.
Available parameters:
- width:
This parameter represents the width of the rectangle which is in pixels.
- Height:
As you know, in addition to the width of the rectangle, it also has a length, which determines the height of the rectangle, and it is also in pixels.
- x:
By using this parameter, you can specify the coordinates that the upper-left corner of the rectangle should have and determine where the rectangle should start from.
- y:
This parameter also specifies the coordinates of the upper-left corner, so with these 4 parameters, a rectangle can be drawn.
Another point that should be mentioned is that you can also specify the color of the rectangle by using the command ctx.strokeStyle, which we are going to show you an example of coding in order to draw a rectangle with the help of JavaScript .
For example, suppose you want to draw a blue rectangle with line width 6, length 250, width 150 and at 5&5 point, you need to do the following with JavaScript.
// Blue rectangle
ctx.beginPath ();
ctx.lineWidth = "6";
ctx.strokeStyle = "Blue";
ctx.rect (5, 5, 250, 150);
ctx.stroke ();
After doing this, you can easily draw a rectangle and use it in order to make your content more attractive.
Now imagine that you want to draw a slightly smaller green rectangle inside this blue rectangle, if you want to do this, you have to do the following.
// Green rectangle
ctx.beginPath ();
ctx.lineWidth = "4";
ctx.strokeStyle = "green";
ctx.rect (30, 30, 70, 50);
ctx.stroke ();
All the things mentioned above is usually done with the help of JavaScript, so in the following we are going to introduce this language to you.
What is JavaScript?
There are different programming languages, one of which is JavaScript, it is a kind of language which different programmers can use in order to implement various features in web pages, another use of it, is in the development of various games , finally all of these enable the user to get a better user experience from using pages and games.
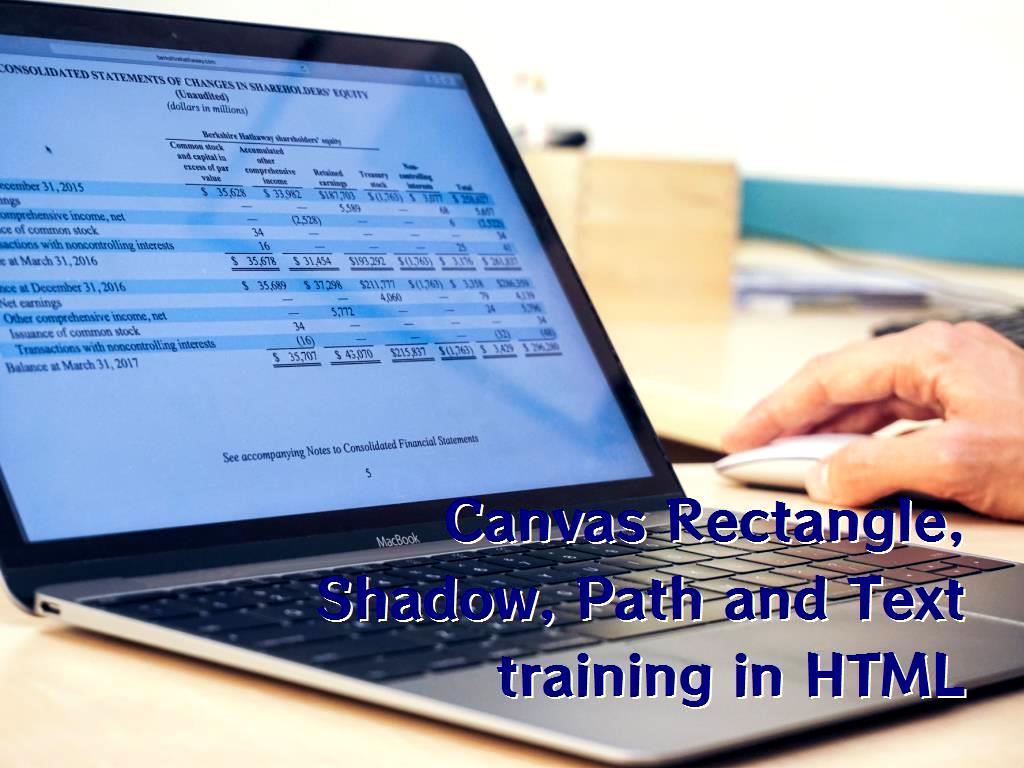
Shadows:
Everyone's effort is to be able to convey a better feeling to the user that in HTML5 canvas you can make images, text, even a line more realistic with the help of shadows, in other words, with the help of shadows, everything can be made 3D, the shadows that are created can have the following properties:
- shadowOffsetX () Property:
This is one of the properties of the shadow which is zero by default, and with help of which you can specify the horizontal distance of the shadow from the shape, it should be also noted that in order to change the position of the shadow, you can use positive or negative values.
The way of doing this is as follows:
ctx.shadowOffsetX = h_distance;
- shadowOffsetY () Property:
This is another property for adding shadow, which is used to adjust the vertical distance of the shadow from the shape, and just like the previous case, it is zero by default, and with the help of positive and negative values, you can determine its position.
In order to do this, you must do the following:
ctx.shadowOffsetX = v_distance;
- shadowBlur () Property:
Another feature for shadows is the amount of blurring that can be adjusted, which you must be done as follows.
ctx.shadowBlur = blur_value
- shadowColor () Property:
Shadows can have different color spectrums which can be adjusted this way: ctx.shadowColor
For example, suppose you want to create several rectangles with the same size which have shadows with different colors in relation to each other, to do this, you can do the following.
<! DOCTYPE html>
<html>
<head> <title> HTML5 Canvas - shadow </title>
</head>
<body>
<canvas id = "DemoCanvas" width = "500" height = "600"> </canvas>
<script>
var canvas = document.getElementById ("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext ('2d');
ctx.shadowColor = "black";
ctx.shadowBlur = 6;
ctx.shadowOffsetX = 6;
ctx.shadowOffsetY = 6;
ctx.shadowColor = "orange";
ctx.strokeRect (25, 25, 300, 200);
ctx.shadowColor = "green";
ctx.strokeRect (50, 50, 300, 200);
ctx.shadowColor = "blue";
ctx.strokeRect (75, 75, 300, 200);
ctx.shadowColor = "red";
ctx.strokeRect (100, 100, 300, 200);
}
</script>
</body>
</html>
In addition to adding a shadow feature to a rectangle or any other shape, you can also add shadows to a text, if you want to make it more attractive to the user.
Path:
In HTML5 canvas, it is possible for you to create custom shapes, for example, imagine that you are going to start drawing a path, first, you need to start from beginPath () and finally draw the path you want, then you need to use the fill () or stroke () methods to create a suitable shape for the user, in the last step you can execute the command of a new path with closePath (), for example imagine that you want to draw a yellow triangle, to do this you have to do the following.
<! DOCTYPE html>
<html>
<head>
<title> Sample arcs example </title>
</head>
<body>
<canvas id = "DemoCanvas" width = "300" height = "600"> </canvas>
<script>
var canvas = document.getElementById ("DemoCanvas");
var context = canvas.getContext ("2d");
// Set the style properties.
context.fillStyle = 'yellow';
context.strokeStyle = 'red';
context.lineWidth = 2;
context.beginPath ();
// Start from the top-left point.
context.moveTo (20, 20); // give the (x, y) coordinates
context.lineTo (190, 20);
context.lineTo (20, 190);
context.lineTo (20, 20);
// Now fill the shape, and draw the stroke.
context.fill ();
context.stroke ();
context.closePath ();
</script>
</body>
</html>
If you want to draw any other path, you can do the same, which may only differ in characteristics, for instance, color, starting point, end point, number of paths, and so on.
Text:
One of the other things that you can do with the help of this version is drawing text, which also has different properties, such as drawing a map, drawing a rectangle, etc., the text may be italics, bold, normal and so on, also the font size and other features may differ from each other, in general, among the characteristics of the texts, the following can be mentioned.
-text:
To draw text on canvas, you can use the string type and draw it.
- x:
To draw text, you must specify what horizontal coordinates you want to draw with the canvas.
- y:
Like drawing all items, you need vertical coordinates for canvas to draw text in addition to horizontal coordinates.
- maxWidth:
Another feature that different texts can have is the maximum text width.
In general, different texts can be drawn, and also different methods can be used to draw each one, which we will mention an example of drawing text with the fillText () method in the following.
If you want to draw the text 'Canvas Rectangle, Shadow, Path' in a simple way, you can do it by coding which is mentioned below.
<! DOCTYPE html>
<html>
<head>
<title> HTML5 Canvas - Text </title>
</head>
<body>
<canvas id = "DemoCanvas" width = "500" height = "600"> </canvas>
<script>
var canvas = document.getElementById ("DemoCanvas");
if (canvas.getContext)
{
var ctx = canvas.getContext ('2d');
ctx.font = 'italic 32px sans-serif';
ctx.fillText ('Canvas Rectangle, Shadow, Path', 10, 50);
}
</script>
</body>
</html>
.jpg)
Last word:
In general, HTML Canvas can be used to draw various items that ultimately cause users to get a better user experience by viewing them, in this article, we have mentioned drawing items, so that it can give you an idea about making your site attractive, as a result, you can increase the traffic to your website with these simple tasks.