In order for local applications to have access to the web, special settings must be made in their design so that they can finally access the web. This article teaches you how to create a class and access the web through the created classes. If you want to know how to create classes in the application and access the web through classes, it is recommended to follow this tutorial.
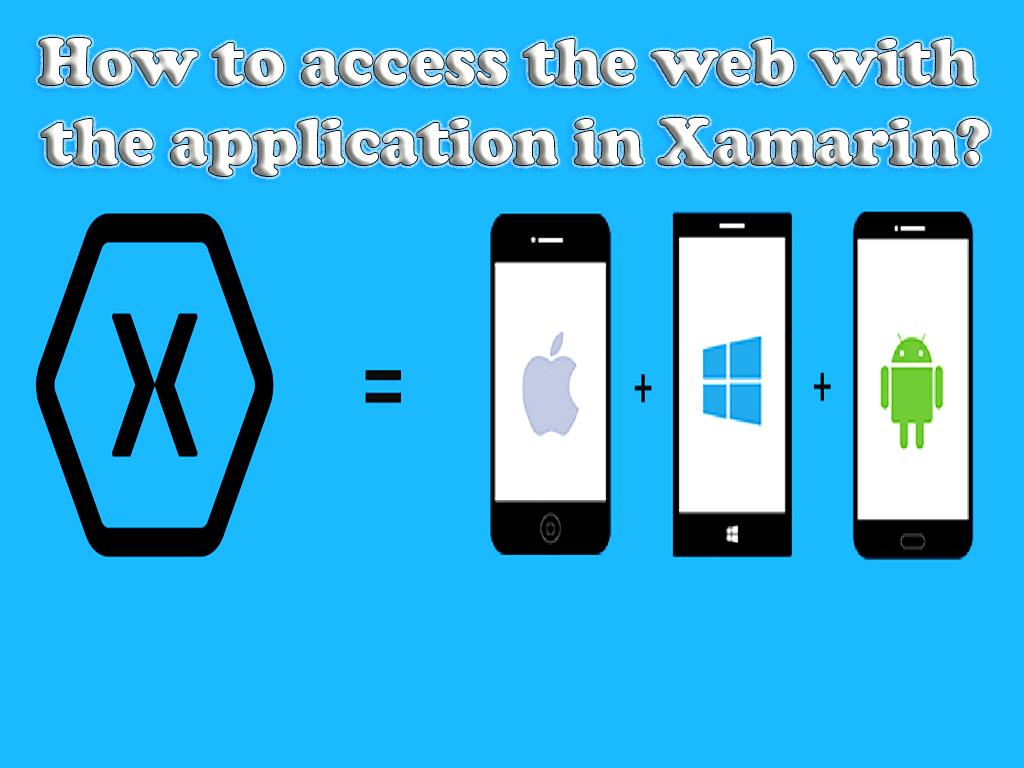
In this section you will learn:
- How to use NuGet Package Manager to add Newtonsoft.Json to Xamarin.Forms projects.
- How to create classes that also have access to the web.
- How to use the classes created to access the web?
The requirements for doing this training are as follows:
- Install the latest version of Visual Studio 2019 on the system
- Install the latest version of Mobile development with .NET on the system
Learn how to add Newtonsoft.Json to Xamarin.Forms projects with NuGet Package Manager using Visual Studio
1- Open Visual Studio.
2- After opening Visual Studio , you need to create a new black Xamarin.Forms app.
3- Choose a name to create. The name chosen in this article is WebServiceTutorial.
4- It is better to be careful in choosing names for the projects and classes created.
Note:
The name chosen for the project must be the same as the name chosen for the solution, so choose the solution name WebServiceTutorial.
5- After naming, you must make sure that the system and application support the .NET Standard mechanism for shared code.
This mechanism is used to share code written in Xamarin.Forms with the C # programming language. If your system and app do not support this mechanism, it cannot have multiple outputs on multiple platforms at once. Therefore, in order to be able to have multiple outputs on several different platforms at the same time, you must be sure that your app and system support this mechanism.
6- Right-click in the Solution Explorer section of the WebServiceTutorial project.
7- Select Manage NuGetPackeges.
8- In the NuGet Packages Manager section, select the Brows tab.
9- Search for Newtonsoft.Json, then install it and add it to the project.
10- Build a Solution to ensure there are no errors.
Learn how to create classes in Xamarin.Forms that also have access to the web using Visual Studio
1. In the Solution Explorer section of the WebServiceTutorial project, create a new class called Constants and add it to the project.
2. Replace all the code listed below with the code in the Constants.cs section.
namespace WebServiceTutorial
{
public static class Constants
{
public const string OpenWeatherMapEndpoint = "Error! Hyperlink reference not valid.";
public const string OpenWeatherMapAPIKey = "INSERT_API_KEY_HERE";
}
}
By entering the above code, you define two constants. OpenWeatherMapEndpoint is an endpoint versus web request, and OpenWeatherMapAPIKey displays your personal API key for OpenWeatherMap.
3. In the Solution Explorer section of the WebServiceTutorial project, create a new class and name it WeatherData and add it to the project.
4. Replace all the codes listed below with the codes in the WeatherData.cs section.
using Newtonsoft.Json;
namespace WebServiceTutorial
{
public class WeatherData
{
[JsonProperty ("name")]
public string Title {get; set;}
[JsonProperty ("weather")]
public Weather [] Weather {get; set;}
[JsonProperty ("main")]
public Main {get; set;}
[JsonProperty ("visibility")]
public long Visibility {get; set;}
[JsonProperty ("wind")]
public Wind {get; set;}
}
public class Main
{
[JsonProperty ("temp")]
public double Temperature {get; set;}
[JsonProperty ("humidity")]
public long Humidity {get; set;}
}
public class Weather
{
[JsonProperty ("main")]
public string Visibility {get; set;}
}
public class Wind
{
[JsonProperty ("speed")]
public double Speed {get; set;}
}
}
By entering this code, you define four classes that are used to model the recovery of JSON data.
5. In the Solution Explorer section of the WebServiceTutorial project, create a new class called RestService and add it to the project.
6. Replace all the codes listed below with the codes in the RestService.cs section.
using System;
using System.Diagnostics;
using System.Net.Http;
using System.Threading. Tasks;
using Newtonsoft.Json;
namespace WebServiceTutorial
{
public class RestService
{
HttpClient _client;
public RestService ()
{
_client = new HttpClient ();
}
public async Task <weatherdata> GetWeatherDataAsync (string uri) {
WeatherData = null;
try
{
HttpResponseMessage response = await _client. GetAsync (uri);
if (response. IsSuccessStatusCode)
{
string content = await response. Content.ReadAsStringAsync ();
weatherData = JsonConvert.DeserializeObject <weatherdata> (content);
}
}
catch (Exception ex)
{
Debug.WriteLine ("\ tERROR {0}", ex. Message);
}
return weatherData;
}
}
}
</weatherdata> </weatherdata>
By entering this code, you define the GetWeatherDataaAsync method. This method uses the HttpClient.GetAsync method to send a GET request to the web API. The web API sends a response that is stored in the HttpResponseMessage object. The response contains the HTTP status code, which is used to determine if the HTTP request was sent successfully.
If the request is successful, the web API will respond with HTTP status code 200 (OK) and JSON response.
7. Build a Solution to ensure that there are no errors.
Learn how to use classes in Xamarin.Forms to access the web using Visual Studio
1- In the Solution Explorer section of the WebServiceTutorial project, double-click on MainPage.xaml.
2- Replace all the codes listed below with the codes in that section.
<! -? xml version = "1.0" encoding = "utf-8"? ->
<contentpage xmlns = "Error! Hyperlink reference not valid." xmlns: x = "Error! Hyperlink reference not valid." x: class = "WebServiceTutorial.MainPage">
<grid margin = "20,35,20,20">
<grid. columndefinitions>
<columndefinition width = "0.4 *">
<columndefinition width = "0.6 *">
</columndefinition> </columndefinition> </grid. columndefinitions>
<grid. rowdefinitions>
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
</rowdefinition></rowdefinition></rowdefinition></rowdefinition></rowdefinition></rowdefinition> </rowdefinition> </grid. rowdefinitions>
<entry x: name = "cityEntry" grid. columnspan = "2" text = "Seattle">
<button grid. columnspan = "2" grid.row = "1" text = "Get Weather" clicked = "OnButtonClicked">
<label grid.row = "2" text = "Location:">
<label grid.row = "2" grid. column = "1" text = "{Binding Title}">
<label grid.row = "3" text = "Temperature:">
<label grid.row = "3" grid. column = "1" text = "{Binding Main.Temperature}">
<label grid.row = "4" text = "Wind Speed:">
<label grid.row = "4" grid. column = "1" text = "{Binding Wind.Speed}">
<label grid.row = "5" text = "Humidity:">
<label grid.row = "5" grid. column = "1" text = "{Binding Main.Humidity}">
<label grid.row = "6" text = "Visibility:">
<label grid.row = "6" grid. column = "1" text = "{Binding Weather [0]. Visibility}">
</label></label></label></label></label></label></label></label></label></label></button> </entry> </ grid> </contentpage>
By entering this code, you specify a UI for the page. This UI includes an Entry, a Button and a number of Labels in the Grid.
3- In the Solution Explorer section of the WebServiceTutorial project, open the MainPage.xaml file.
4- Double click on MainPage.xaml.cs and replace all the codes listed below with the codes in that file.
using System;
using Xamarin.Forms;
namespace WebServiceTutorial
{
public partial class MainPage: ContentPage
{
RestService _restService;
public MainPage ()
{
InitializeComponent ();
_restService = new RestService ();
}
async void OnButtonClicked (object sender, EventArgs e)
{
if (! string. IsNullOrWhiteSpace (cityEntry.Text))
{
WeatherData = await _restService.GetWeatherDataAsync (GenerateRequestUri (Constants.OpenWeatherMapEndpoint));
BindingContext = weatherData;
}
}
string GenerateRequestUri (string endpoint)
{
string requestUri = endpoint;
requestUri + = $ "? q = {cityEntry.Text}";
requestUri + = "& units = imperial"; // or units = metric
requestUri + = $ "& APPID = {Constants. OpenWeatherMapAPIKey}";
return requestUri;
}
}
}
The OnButtonClicked method is executed when the Button is hit.
5- Press the start button or the Ctrl + F5 key combination to see the result of the applied changes.
The requirements for doing this training are as follows:
- Install the latest version of Visual Studio for Mac on the system
- Install the latest version of support platform for Android and iOS on the system
- Install the latest version of Xcode on the system
Learn how to add Newtonsoft.Json to Xamarin.Forms projects with NuGet Package Manager using Visual Studio for Mac
1- Open Visual Studio for Mac.
2- After opening Visual Studio for Mac, you need to create a new black Xamarin.Forms app .
3- Choose a name to create. The name chosen in this article is WebServiceTutorial.
4- Note: It is better to be careful in choosing names for the projects and classes created.
Note:
The name chosen for the project must be the same as the name chosen for the solution, so choose the solution name WebServiceTutorial.
5- After naming, you must make sure that the system and application support the .NET Standard mechanism for shared code.
This mechanism is used to share code written in Xamarin.Forms with the C # programming language. If your system and app do not support this mechanism, it cannot have multiple outputs on multiple platforms at once. Therefore, in order to be able to have multiple outputs on several different platforms at the same time, you must be sure that your app and system support this mechanism.
6- Right-click on the Solution pad of the WebServiceTutorial project.
7- Select Manage NuGetPackeges.
8- In the Newtonsoft.Json window, search for Add Packages, then install it and add it to the project.
9- Build a Solution to ensure there are no errors.
Learn how to create classes in Xamarin.Forms that also have web access using Visual Studio for Mac
1- In the Solution pad section of the WebServiceTutorial project, create a new class called Constants and add it to the project.
2- Replace all the code listed below with the code in the Constants.cs section.
namespace WebServiceTutorial
{
public static class Constants
{
public const string OpenWeatherMapEndpoint = "Error! Hyperlink reference not valid.";
public const string OpenWeatherMapAPIKey = "INSERT_API_KEY_HERE";
}
}
By entering the above code, you define two constants. OpenWeatherMapEndpoint is an endpoint versus web request, and OpenWeatherMapAPIKey displays your personal API key for OpenWeatherMap.
3- In the Solution Explorer section of the WebServiceTutorial project, create a new class and name it WeatherData and add it to the project.
4- Replace all the codes listed below with the codes in the WeatherData.cs section.
using Newtonsoft.Json;
namespace WebServiceTutorial
{
public class WeatherData
{
[JsonProperty ("name")]
public string Title {get; set;}
[JsonProperty ("weather")]
public Weather [] Weather {get; set;}
[JsonProperty ("main")]
public Main {get; set;}
[JsonProperty ("visibility")]
public long Visibility {get; set;}
[JsonProperty ("wind")]
public Wind {get; set;}
}
public class Main
{
[JsonProperty ("temp")]
public double Temperature {get; set;}
[JsonProperty ("humidity")]
public long Humidity {get; set;}
}
public class Weather
{
[JsonProperty ("main")]
public string Visibility {get; set;}
}
public class Wind
{
[JsonProperty ("speed")]
public double Speed {get; set;}
}
}
By entering this code, you define four classes that are used to model the recovery of JSON data.
5- In the Solution pad section of the WebServiceTutorial project, create a new class and name it RestService and add it to the project.
6- Replace all the codes listed below with the codes in the RestService.cs section.
using System;
using System.Diagnostics;
using System.Net.Http;
using System.Threading. Tasks;
using Newtonsoft.Json;
namespace WebServiceTutorial
{
public class RestService
{
HttpClient _client;
public RestService ()
{
_client = new HttpClient ();
}
public async Task <weatherdata> GetWeatherDataAsync (string uri)
{
WeatherData = null;
try
{
HttpResponseMessage response = await _client. GetAsync (uri);
if (response. IsSuccessStatusCode)
{
string content = await response. Content.ReadAsStringAsync ();
weatherData = JsonConvert.DeserializeObject <weatherdata> (content);
}
}
catch (Exception ex)
{
Debug.WriteLine ("\ tERROR {0}", ex. Message);
}
Return entering this code, you define the GetWeatherDataaAsync method. This method uses the HttpClient.GetAsync method to send a GET request to the web API. The web API sends a response that is stored in the HttpResponseMessage object. The response contains the HTTP status code, which is used to determine if the HTTP request was sent successfully.
If the request is successful, the web API will respond with HTTP status code 200 (OK) and JSON response.
7- Build a Solution to ensure that there are no errors.
Learn how to use classes in Xamarin.Forms to access the web using Visual Studio for Mac
1- Double click on MainPage.xaml in the Solution pad of the WebServiceTutorial project.
2- Replace all the codes listed below with the codes in that section.
<! -? xml version = "1.0" encoding = "utf-8"? ->
<contentpage xmlns = "Error! Hyperlink reference not valid." xmlns: x = "Error! Hyperlink reference not valid." x: class = "WebServiceTutorial.MainPage">
<grid margin = "20,35,20,20">
<grid. columndefinitions>
<columndefinition width = "0.4 *">
<columndefinition width = "0.6 *">
</columndefinition> </columndefinition> </grid. columndefinitions>
<grid. rowdefinitions>
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
<rowdefinition height = "40">
</rowdefinition></rowdefinition></rowdefinition></rowdefinition></rowdefinition></rowdefinition> </rowdefinition> </grid. rowdefinitions>
<entry x: name = "cityEntry" grid. columnspan = "2" text = "Seattle">
<button grid. columnspan = "2" grid.row = "1" text = "Get Weather" clicked = "OnButtonClicked">
<label grid.row = "2" text = "Location:">
<label grid.row = "2" grid. column = "1" text = "{Binding Title}">
<label grid.row = "3" text = "Temperature:">
<label grid.row = "3" grid. column = "1" text = "{Binding Main.Temperature}">
<label grid.row = "4" text = "Wind Speed:">
<label grid.row = "4" grid. column = "1" text = "{Binding Wind.Speed}">
<label grid.row = "5" text = "Humidity:">
<label grid.row = "5" grid. column = "1" text = "{Binding Main.Humidity}">
<label grid.row = "6" text = "Visibility:">
<label grid.row = "6" grid. column = "1" text = "{Binding Weather [0]. Visibility}">
</label></label></label></label></label></label></label></label></label></label></button> </entry> </ grid> </contentpage>
By entering this code, you specify a UI for the page. This UI includes an Entry, a Button and a number of Labels in the Grid.
3- In the Solution Explorer section of the WebServiceTutorial project, open the MainPage.xaml file.
4- Double click on MainPage.xaml.cs and replace all the codes listed below with the codes in that file.
using System;
using Xamarin.Forms;
namespace WebServiceTutorial
{
public partial class MainPage: ContentPage
{
RestService _restService;
public MainPage ()
{
InitializeComponent ();
_restService = new RestService ();
}
async void OnButtonClicked (object sender, EventArgs e)
{
if (! string. IsNullOrWhiteSpace (cityEntry.Text))
{
WeatherData = await _restService.GetWeatherDataAsync (GenerateRequestUri (Constants.OpenWeatherMapEndpoint));
BindingContext = weatherData;
}
}
string GenerateRequestUri (string endpoint)
{
string requestUri = endpoint;
requestUri + = $ "? q = {cityEntry.Text}";
requestUri + = "& units = imperial"; // or units = metric
requestUri + = $ "& APPID = {Constants.OpenWeatherMapAPIKey}";
return requestUri;
}
}
}
5- The OnButtonClicked method is executed when the Button is hit.
6- Press the start button or the Ctrl + F5 key combination to see the result of the applied changes.
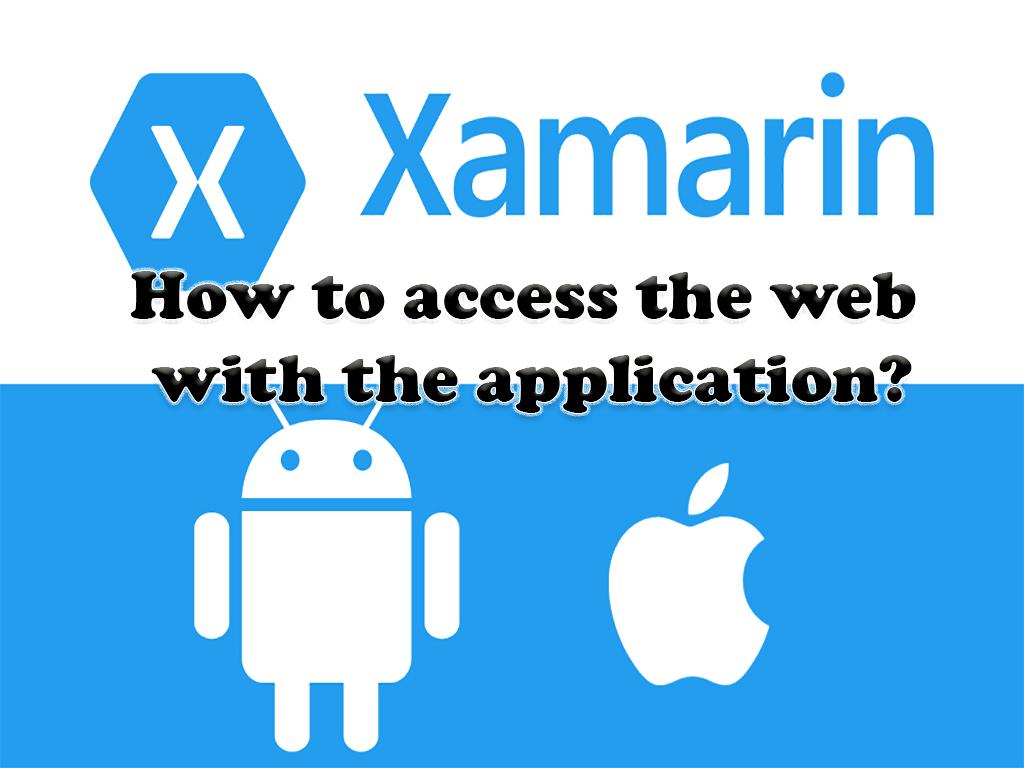
Finally, you were able to do the following:
- How to use NuGet Package Manager to add Newtonsoft.Json to Xamarin.Forms projects.
- How to create classes that also have access to the web.
- How to use the classes created to access the web.